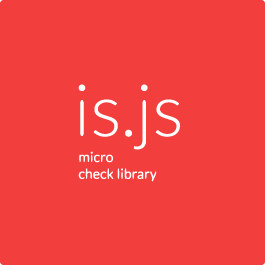
Check types, regexps, presence, time and more...
View on GitHubType
- arguments
- array
- boolean
- date
- error
- function
- nan
- null
- number
- object
- json
- regexp
- string
- char
- undefined
- sameType
Presence
RegExp
- url
- creditCard
- alphaNumeric
- timeString
- dateString
- usZipCode
- caPostalCode
- ukPostCode
- nanpPhone
- eppPhone
- socialSecurityNumber
- affirmative
- hexadecimal
- hexColor
- ip
- ipv4
- ipv6
String
Arithmetic
Object
Array
Environment
type checks
is.arguments(value:any)
interfaces: not, all, anyChecks if the given value type is arguments.
var getArguments = function() {
return arguments;
};
var arguments = getArguments();
is.arguments(arguments);
=> true
is.not.arguments({foo: 'bar'});
=> true
is.all.arguments(arguments, 'bar');
=> false
is.any.arguments(['foo'], arguments);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.arguments([arguments, 'foo', 'bar']);
=> false
is.array(value:any)
interfaces: not, all, anyChecks if the given value type is array.
is.array(['foo', 'bar', 'baz']);
=> true
is.not.array({foo: 'bar'});
=> true
is.all.array(['foo'], 'bar');
=> false
is.any.array(['foo'], 'bar');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.array([[1, 2], 'foo', 'bar']);
=> false
is.boolean(value:any)
interfaces: not, all, anyChecks if the given value type is boolean.
is.boolean(true);
=> true
is.not.boolean({foo: 'bar'});
=> true
is.all.boolean(true, 'bar');
=> false
is.any.boolean(true, 'bar');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.boolean([true, 'foo', 'bar']);
=> false
is.date(value:any)
interfaces: not, all, anyChecks if the given value type is date.
is.date(new Date());
=> true
is.not.date({foo: 'bar'});
=> true
is.all.date(new Date(), 'bar');
=> false
is.any.date(new Date(), 'bar');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.date([new Date(), 'foo', 'bar']);
=> false
is.error(value:any)
interfaces: not, all, anyChecks if the given value type is error.
is.error(new Error());
=> true
is.not.error({foo: 'bar'});
=> true
is.all.error(new Error(), 'bar');
=> false
is.any.error(new Error(), 'bar');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.error([new Error(), 'foo', 'bar']);
=> false
is.function(value:any)
interfaces: not, all, anyChecks if the given value type is function.
is.function(toString);
=> true
is.not.function({foo: 'bar'});
=> true
is.all.function(toString, 'bar');
=> false
is.any.function(toString, 'bar');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.function([toString, 'foo', 'bar']);
=> false
is.nan(value:any)
interfaces: not, all, anyChecks if the given value type is NaN.
is.nan(NaN);
=> true
is.not.nan(42);
=> true
is.all.nan(NaN, 1);
=> false
is.any.nan(NaN, 2);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.nan([NaN, 'foo', 1]);
=> false
is.null(value:any)
interfaces: not, all, anyChecks if the given value type is null.
is.null(null);
=> true
is.not.null(42);
=> true
is.all.null(null, 1);
=> false
is.any.null(null, 2);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.null([null, 'foo', 1]);
=> false
is.number(value:any)
interfaces: not, all, anyChecks if the given value type is number.
is.number(42);
=> true
is.number(NaN);
=> false
is.not.number('42');
=> true
is.all.number('foo', 1);
=> false
is.any.number({}, 2);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.number([42, 'foo', 1]);
=> false
is.object(value:any)
interfaces: not, all, anyChecks if the given value type is object.
is.object({foo: 'bar'});
=> true
// functions are also returnin as true
is.object(toString);
=> true
is.not.object('foo');
=> true
is.all.object({}, 1);
=> false
is.any.object({}, 2);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.object([{}, new Object()]);
=> true
is.json(value:any)
interfaces: not, all, anyChecks if the given value type is pure json object.
is.json({foo: 'bar'});
=> true
// functions are returning as false
is.json(toString);
=> false
is.not.json([]);
=> true
is.all.json({}, 1);
=> false
is.any.json({}, 2);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.json([{}, {foo: 'bar'}]);
=> true
is.regexp(value:any)
interfaces: not, all, anyChecks if the given value type is RegExp.
is.regexp(/test/);
=> true
is.not.regexp(['foo']);
=> true
is.all.regexp(/test/, 1);
=> false
is.any.regexp(new RegExp('ab+c'), 2);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.regexp([{}, /test/]);
=> false
is.string(value:any)
interfaces: not, all, anyChecks if the given value type is string.
is.string('foo');
=> true
is.not.string(['foo']);
=> true
is.all.string('foo', 1);
=> false
is.any.string('foo', 2);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.string([{}, 'foo']);
=> false
is.char(value:any)
interfaces: not, all, anyChecks if the given value type is char.
is.char('f');
=> true
is.not.char(['foo']);
=> true
is.all.char('f', 1);
=> false
is.any.char('f', 2);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.char(['f', 'o', 'o']);
=> true
is.undefined(value:any)
interfaces: not, all, anyChecks if the given value type is undefined.
is.undefined(undefined);
=> true
is.not.undefined(null);
=> true
is.all.undefined(undefined, 1);
=> false
is.any.undefined(undefined, 2);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.undefined([{}, undefined]);
=> false
is.sameType(value:any, value:any)
interface: notChecks if the given value types are same.
is.sameType(42, 7);
=> true
is.sameType(42, '7');
=> false
is.not.sameType(42, 7);
=> false
presence checks
is.empty(value:object|array|string)
interfaces: not, all, anyChecks if the given value is empty.
is.empty({});
=> true
is.empty([]);
=> true
is.empty('');
=> true
is.not.empty(['foo']);
=> true
is.all.empty('', {}, ['foo']);
=> false
is.any.empty([], 42);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.empty([{}, 'foo']);
=> false
is.existy(value:any)
interfaces: not, all, anyChecks if the given value is existy. (not null or undefined)
is.existy({});
=> true
is.existy(null);
=> false
is.not.existy(undefined);
=> true
is.all.existy(null, ['foo']);
=> false
is.any.existy(undefined, 42);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.existy([{}, 'foo']);
=> true
is.truthy(value:any)
interfaces: not, all, anyChecks if the given value is truthy. (existy and not false)
is.truthy(true);
=> true
is.truthy(null);
=> false
is.not.truthy(false);
=> true
is.all.truthy(null, true);
=> false
is.any.truthy(undefined, true);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.truthy([{}, true]);
=> true
is.falsy(value:any)
interfaces: not, all, anyChecks if the given value is falsy.
is.falsy(false);
=> true
is.falsy(null);
=> true
is.not.falsy(true);
=> true
is.all.falsy(null, false);
=> true
is.any.falsy(undefined, true);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.falsy([false, true, undefined]);
=> false
is.space(value:string)
interfaces: not, all, anyChecks if the given value is space.
is.space(' ');
=> true
is.space('foo');
=> false
is.not.space(true);
=> true
is.all.space(' ', 'foo');
=> false
is.any.space(' ', true);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.space([' ', 'foo', undefined]);
=> false
regexp checks
is.url(value:any)
interfaces: not, all, anyChecks if the given value matches url regexp.
is.url('http://www.test.com');
=> true
is.url('foo');
=> false
is.not.url(true);
=> true
is.all.url('http://www.test.com', 'foo');
=> false
is.any.url('http://www.test.com', true);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.url(['http://www.test.com', 'foo', undefined]);
=> false
is.email(value:any)
interfaces: not, all, anyChecks if the given value matches email regexp.
is.email('test@test.com');
=> true
is.email('foo');
=> false
is.not.email('foo');
=> true
is.all.email('test@test.com', 'foo');
=> false
is.any.email('test@test.com', 'foo');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.email(['test@test.com', 'foo', undefined]);
=> false
is.creditCard(value:any)
interfaces: not, all, anyChecks if the given value matches credit card regexp.
is.creditCard(378282246310005);
=> true
is.creditCard(123);
=> false
is.not.creditCard(123);
=> true
is.all.creditCard(378282246310005, 123);
=> false
is.any.creditCard(378282246310005, 123);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.creditCard([378282246310005, 123, undefined]);
=> false
is.alphaNumeric(value:any)
interfaces: not, all, anyChecks if the given value matches alpha numeric regexp.
is.alphaNumeric('alphaNu3er1k');
=> true
is.alphaNumeric('*?');
=> false
is.not.alphaNumeric('*?');
=> true
is.all.alphaNumeric('alphaNu3er1k', '*?');
=> false
is.any.alphaNumeric('alphaNu3er1k', '*?');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.alphaNumeric(['alphaNu3er1k', '*?']);
=> false
is.timeString(value:any)
interfaces: not, all, anyChecks if the given value matches time string regexp.
is.timeString('13:45:30');
=> true
is.timeString('90:90:90');
=> false
is.not.timeString('90:90:90');
=> true
is.all.timeString('13:45:30', '90:90:90');
=> false
is.any.timeString('13:45:30', '90:90:90');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.timeString(['13:45:30', '90:90:90']);
=> false
is.dateString(value:any)
interfaces: not, all, anyChecks if the given value matches date string regexp.
is.dateString('11/11/2011');
=> true
is.dateString('90/11/2011');
=> false
is.not.dateString('90/11/2011');
=> true
is.all.dateString('11/11/2011', '90/11/2011');
=> false
is.any.dateString('11/11/2011', '90/11/2011');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.dateString(['11/11/2011', '90/11/2011']);
=> false
is.usZipCode(value:any)
interfaces: not, all, anyChecks if the given value matches US zip code regexp.
is.usZipCode('02201-1020');
=> true
is.usZipCode('123');
=> false
is.not.usZipCode('123');
=> true
is.all.usZipCode('02201-1020', '123');
=> false
is.any.usZipCode('02201-1020', '123');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.usZipCode(['02201-1020', '123']);
=> false
is.caPostalCode(value:any)
interfaces: not, all, anyChecks if the given value matches Canada postal code regexp.
is.caPostalCode('L8V3Y1');
=> true
is.caPostalCode('123');
=> false
is.not.caPostalCode('123');
=> true
is.all.caPostalCode('L8V3Y1', '123');
=> false
is.any.caPostalCode('L8V3Y1', '123');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.caPostalCode(['L8V3Y1', '123']);
=> false
is.ukPostCode(value:any)
interfaces: not, all, anyChecks if the given value matches UK post code regexp.
is.ukPostCode('B184BJ');
=> true
is.ukPostCode('123');
=> false
is.not.ukPostCode('123');
=> true
is.all.ukPostCode('B184BJ', '123');
=> false
is.any.ukPostCode('B184BJ', '123');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.ukPostCode(['B184BJ', '123']);
=> false
is.nanpPhone(value:any)
interfaces: not, all, anyChecks if the given value matches North American numbering plan phone regexp.
is.nanpPhone('609-555-0175');
=> true
is.nanpPhone('123');
=> false
is.not.nanpPhone('123');
=> true
is.all.nanpPhone('609-555-0175', '123');
=> false
is.any.nanpPhone('609-555-0175', '123');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.nanpPhone(['609-555-0175', '123']);
=> false
is.eppPhone(value:any)
interfaces: not, all, anyChecks if the given value matches extensible provisioning protocol phone regexp.
is.eppPhone('+90.2322456789');
=> true
is.eppPhone('123');
=> false
is.not.eppPhone('123');
=> true
is.all.eppPhone('+90.2322456789', '123');
=> false
is.any.eppPhone('+90.2322456789', '123');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.eppPhone(['+90.2322456789', '123']);
=> false
is.affirmative(value:any)
interfaces: not, all, anyChecks if the given value matches affirmative regexp.
is.affirmative('yes');
=> true
is.affirmative('no');
=> false
is.not.affirmative('no');
=> true
is.all.affirmative('yes', 'no');
=> false
is.any.affirmative('yes', 'no');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.affirmative(['yes', 'y', 'true', 't', 'ok', 'okay']);
=> true
is.hexadecimal(value:any)
interfaces: not, all, anyChecks if the given value matches hexadecimal regexp.
is.hexadecimal('f0f0f0');
=> true
is.hexadecimal(2.5);
=> false
is.not.hexadecimal('string');
=> true
is.all.hexadecimal('ff', 'f50');
=> true
is.any.hexadecimal('ff5500', true);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.hexadecimal(['fff', '333', 'f50']);
=> true
is.hexColor(value:any)
interfaces: not, all, anyChecks if the given value matches hexColor regexp.
is.hexColor('#333');
=> true
is.hexColor('#3333');
=> false
is.not.hexColor(0.5);
=> true
is.all.hexColor('fff', 'f50');
=> true
is.any.hexColor('ff5500', 0.5);
=> false
// 'all' and 'any' interfaces can also take array parameter
is.all.hexColor(['fff', '333', 'f50']);
=> true
is.ip(value:any)
interfaces: not, all, anyChecks if the given value matches ip regexp.
is.ip('198.156.23.5');
=> true
is.ip('1.2..5');
=> false
is.not.ip('8:::::::7');
=> true
is.all.ip('0:1::4:ff5:54:987:C', '123.123.123.123');
=> true
is.any.ip('123.8.4.3', '0.0.0.0');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.ip(['123.123.23.12', 'A:B:C:D:E:F:0:0']);
=> true
is.ipv4(value:any)
interfaces: not, all, anyChecks if the given value matches ipv4 regexp.
is.ipv4('198.12.3.142');
=> true
is.ipv4('1.2..5');
=> false
is.not.ipv4('8:::::::7');
=> true
is.all.ipv4('198.12.3.142', '123.123.123.123');
=> true
is.any.ipv4('255.255.255.255', '850..1.4');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.ipv4(['198.12.3.142', '1.2.3']);
=> false
is.ipv6(value:any)
interfaces: not, all, anyChecks if the given value matches ipv6 regexp.
is.ipv6('2001:DB8:0:0:1::1');
=> true
is.ipv6('985.12.3.4');
=> true
is.not.ipv6('8:::::::7');
=> true
is.all.ipv6('2001:DB8:0:0:1::1', '1:50:198:2::1:2:8');
=> true
is.any.ipv6('255.255.255.255', '2001:DB8:0:0:1::1');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.ipv6(['2001:DB8:0:0:1::1', '1.2.3']);
=> false
string checks
is.include(value:string, value:substring)
interface: notChecks if the given string contains a substring.
is.include('Some text goes here', 'text');
=> true
is.include('test', 'text');
=> false
is.not.include('test', 'text');
=> true
is.upperCase(value:string)
interfaces: not, all, anyChecks if the given string is UPPERCASE.
is.upperCase('YEAP');
=> true
is.upperCase('nope');
=> false
is.not.upperCase('Nope');
=> true
is.all.upperCase('YEAP', 'nope');
=> false
is.any.upperCase('YEAP', 'nope');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.upperCase(['YEAP', 'ALL UPPERCASE']);
=> true
is.lowerCase(value:string)
interfaces: not, all, anyChecks if the given string is lowercase.
is.lowerCase('yeap');
=> true
is.lowerCase('NOPE');
=> false
is.not.lowerCase('Nope');
=> true
is.all.lowerCase('yeap', 'NOPE');
=> false
is.any.lowerCase('yeap', 'NOPE');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.lowerCase(['yeap', 'all lowercase']);
=> true
is.startWith(value:string, value:substring)
interface: notChecks if the given string starts with substring.
is.startWith('yeap', 'ye');
=> true
is.startWith('nope', 'ye');
=> false
is.not.startWith('nope not that', 'not');
=> true
is.endWith(value:string, value:substring)
interfaces: notChecks if the given string ends with substring.
is.endWith('yeap', 'ap');
=> true
is.endWith('nope', 'no');
=> false
is.not.endWith('nope not that', 'not');
=> true
is.endWith('yeap that one', 'one');
=> true
is.capitalized(value:string)
interfaces: not, all, anyChecks if the given string is capitalized.
is.capitalized('Yeap');
=> true
is.capitalized('nope');
=> false
is.not.capitalized('nope not capitalized');
=> true
is.capitalized('Yeap Capitalized');
=> true
is.all.capitalized('Yeap', 'All', 'Capitalized');
=> true
is.any.capitalized('Yeap', 'some', 'Capitalized');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.capitalized(['Nope', 'not']);
=> false
is.palindrome(value:string)
interfaces: not, all, anyChecks if the given string is palindrome.
is.palindrome('testset');
=> true
is.palindrome('nope');
=> false
is.not.palindrome('nope not palindrome');
=> true
is.not.palindrome('tt');
=> false
is.all.palindrome('testset', 'tt');
=> true
is.any.palindrome('Yeap', 'some', 'testset');
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.palindrome(['Nope', 'testset']);
=> false
arithmetic checks
is.equal(value:any, value:any)
interfaces: notChecks if the given values are equal.
is.equal(42, 40 + 2);
=> true
is.equal('yeap', 'yeap');
=> true
is.equal(true, true);
=> true
is.not.equal('yeap', 'nope');
=> true
is.even(value:number)
interfaces: not, all, anyChecks if the given value is even.
is.even(42);
=> true
is.not.even(41);
=> true
is.all.even(40, 42, 44);
=> true
is.any.even(39, 42, 43);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.even([40, 42, 43]);
=> false
is.odd(value:number)
interfaces: not, all, anyChecks if the given value is odd.
is.odd(41);
=> true
is.not.odd(42);
=> true
is.all.odd(39, 41, 43);
=> true
is.any.odd(39, 42, 44);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.odd([40, 42, 43]);
=> false
is.positive(value:number)
interfaces: not, all, anyChecks if the given value is positive.
is.positive(41);
=> true
is.not.positive(-42);
=> true
is.all.positive(39, 41, 43);
=> true
is.any.positive(-39, 42, -44);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.positive([40, 42, -43]);
=> false
is.negative(value:number)
interfaces: not, all, anyChecks if the given value is negative.
is.negative(-41);
=> true
is.not.negative(42);
=> true
is.all.negative(-39, -41, -43);
=> true
is.any.negative(-39, 42, 44);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.negative([40, 42, -43]);
=> false
is.above(value:number, min)
interface: notChecks if the given value is above minimum value.
is.above(41, 30);
=> true
is.not.above(42, 50);
=> true
is.under(value:number, max)
interface: notChecks if the given value is under maximum value.
is.under(30, 35);
=> true
is.not.under(42, 30);
=> true
is.within(value:number, min, max)
interface: notChecks if the given value is within minimum and maximum values.
is.within(30, 20, 40);
=> true
is.not.within(40, 30, 35);
=> true
is.decimal(value:number)
interfaces: not, all, anyChecks if the given value is decimal.
is.decimal(41.5);
=> true
is.not.decimal(42);
=> true
is.all.decimal(39.5, 41.5, -43.5);
=> true
is.any.decimal(-39, 42.5, 44);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.decimal([40, 42.5, -43]);
=> false
is.integer(value:number)
interfaces: not, all, anyChecks if the given value is integer.
is.integer(41);
=> true
is.not.integer(42.5);
=> true
is.all.integer(39, 41, -43);
=> true
is.any.integer(-39, 42.5, 44);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.integer([40, 42.5, -43]);
=> false
is.finite(value:number)
interfaces: not, all, anyChecks if the given value is finite.
is.finite(41);
=> true
is.not.finite(42 / 0);
=> true
is.all.finite(39, 41, -43);
=> true
is.any.finite(-39, Infinity, 44);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.finite([Infinity, -Infinity, 42.5]);
=> false
is.infinite(value:number)
interfaces: not, all, anyChecks if the given value is infinite.
is.infinite(Infinity);
=> true
is.not.infinite(42);
=> true
is.all.infinite(Infinity, -Infinity, -43 / 0);
=> true
is.any.infinite(-39, Infinity, 44);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.infinite([Infinity, -Infinity, 42.5]);
=> false
object checks
is.propertyCount(value:object, count)
interface: notChecks if the objects' property count is equal to given count.
is.propertyCount({this: 'is', 'sample': object}, 2);
=> true
is.propertyCount({this: 'is', 'sample': object}, 3);
=> false
is.not.propertyCount({}, 2);
=> true
is.propertyDefined(value:object, property)
interface: notChecks if the given property is defined on object.
is.propertyDefined({yeap: 'yeap'}, 'yeap');
=> true
is.propertyDefined({yeap: 'yeap'}, 'nope');
=> false
is.not.propertyDefined({}, 'nope');
=> true
is.windowObject(value:window)
interface: not, all, anyChecks if the given object is window object.
is.windowObject(window);
=> true
is.windowObject({nope: 'nope'});
=> false
is.not.windowObject({});
=> true
is.all.windowObject(window, {nope: 'nope'});
=> false
is.any.windowObject(window, {nope: 'nope'});
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.windowObject([window, {nope: 'nope'}]);
=> false
is.domNode(value:object)
interface: not, all, anyChecks if the given object is a dom node.
var obj = document.createElement('div');
is.domNode(obj);
=> true
is.domNode({nope: 'nope'});
=> false
is.not.domNode({});
=> true
is.all.domNode(obj, obj);
=> true
is.any.domNode(obj, {nope: 'nope'});
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.domNode([obj, {nope: 'nope'}]);
=> false
array check
is.inArray(value:array)
interface: notChecks if the given item is in array.
is.inArray(2, [1, 2, 3]);
=> true
is.inArray(4, [1, 2, 3]);
=> false
is.not.inArray(4, [1, 2, 3]);
=> true
is.sorted(value:array)
interfaces: not, all, anyChecks if the given array is sorted.
is.sorted([1, 2, 3]);
=> true
is.sorted([1, 2, 4, 3]);
=> false
is.not.sorted([5, 4, 3]);
=> true
is.all.sorted([1, 2], [3, 4]);
=> true
is.any.sorted([1, 2], [5, 4]);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.sorted([[1, 2], [5, 4]]);
=> false
environment checks
Environment checks are not available as node module.
is.ie(value:number)
interface: notChecks if the current browser is ie. Parameter is optional version number of browser.
is.ie();
=> true if current browser is ie
is.ie(6);
=> hopefully false
is.not.ie();
=> false if current browser is ie
is.edge()
interface: notChecks if the current browser is edge.
is.edge();
=> true if current browser is edge
is.not.edge();
=> false if current browser is edge
is.chrome()
interface: notChecks if the current browser is chrome.
is.chrome();
=> true if current browser is chrome
is.not.chrome();
=> false if current browser is chrome
is.firefox()
interface: notChecks if the current browser is firefox.
is.firefox();
=> true if current browser is firefox
is.not.firefox();
=> false if current browser is firefox
is.opera()
interface: notChecks if the current browser is opera.
is.opera();
=> true if current browser is opera
is.not.opera();
=> false if current browser is opera
is.safari()
interface: notChecks if the current browser is safari.
is.safari();
=> true if current browser is safari
is.not.safari();
=> false if current browser is safari
is.ios()
interface: notChecks if the current device has ios.
is.ios();
=> true if current device is iPhone, iPad or iPod
is.not.ios();
=> true if current device is not iPhone, iPad or iPod
is.iphone()
interface: notChecks if the current device is iPhone.
is.iphone();
=> true if current device is iPhone
is.not.iphone();
=> true if current device is not iPhone
is.ipad()
interface: notChecks if the current device is iPad.
is.ipad();
=> true if current device is iPad
is.not.ipad();
=> true if current device is not iPad
is.ipod()
interface: notChecks if the current device is iPod.
is.ipod();
=> true if current device is iPod
is.not.ipod();
=> true if current device is not iPod
is.android()
interface: notChecks if the current device has Android.
is.android();
=> true if current device has Android OS
is.not.android();
=> true if current device has not Android OS
is.androidPhone()
interface: notChecks if the current device is Android phone.
is.androidPhone();
=> true if current device is Android phone
is.not.androidPhone();
=> true if current device is not Android phone
is.androidTablet()
interface: notChecks if the current device is Android tablet.
is.androidTablet();
=> true if current device is Android tablet
is.not.androidTablet();
=> true if current device is not Android tablet
is.blackberry()
interface: notChecks if the current device is Blackberry.
is.blackberry();
=> true if current device is Blackberry
is.not.blackberry();
=> true if current device is not Blackberry
is.windowsPhone()
interface: notChecks if the current device is Windows phone.
is.windowsPhone();
=> true if current device is Windows phone
is.not.windowsPhone();
=> true if current device is not Windows Phone
is.windowsTablet()
interface: notChecks if the current device is Windows tablet.
is.windowsTablet();
=> true if current device is Windows tablet
is.not.windowsTablet();
=> true if current device is not Windows tablet
is.windows()
interface: notChecks if the current OS is Windows.
is.windows();
=> true if current OS is Windows
is.not.windows();
=> true if current OS is not Windows
is.mac()
interface: notChecks if the current OS is Mac OS X.
is.mac();
=> true if current OS is Mac OS X
is.not.mac();
=> true if current OS is not Mac OS X
is.linux()
interface: notChecks if the current OS is linux.
is.linux();
=> true if current OS is linux
is.not.linux();
=> true if current OS is not linux
is.desktop()
interface: notChecks if the current device is desktop.
is.desktop();
=> true if current device is desktop
is.not.desktop();
=> true if current device is not desktop
is.mobile()
interface: notChecks if the current device is mobile.
iPhone, iPod, Android Phone, Windows Phone, Blackberry.
is.mobile();
=> true if current device is mobile
is.not.mobile();
=> true if current device is not mobile
is.tablet()
interface: notChecks if the current device is tablet.
iPad, Android Tablet, Windows Tablet
is.tablet();
=> true if current device is tablet
is.not.tablet();
=> true if current device is not tablet
is.online()
interface: notChecks if the current device is online.
is.online();
=> true if current device is online
is.not.online();
=> true if current device is not online
is.offline()
interface: notChecks if the current device is offline.
is.offline();
=> true if current device is offline
is.not.offline();
=> true if current device is not offline
is.touchDevice()
interface: notChecks if the current device supports touch.
is.touchDevice();
=> true if current device supports touch
is.not.touchDevice();
=> true if current device doesn't support touch
time checks
is.today(value:object)
interfaces: not, all, anyChecks if the given date object indicate today.
var today = new Date();
is.today(today);
=> true
var yesterday = new Date(new Date().setDate(new Date().getDate() - 1));
is.today(yesterday);
=> false
is.not.today(yesterday);
=> true
is.all.today(today, today);
=> true
is.any.today(today, yesterday);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.today([today, yesterday]);
=> false
is.yesterday(value:object)
interfaces: not, all, anyChecks if the given date object indicate yesterday.
var today = new Date();
is.yesterday(today);
=> false
var yesterday = new Date(new Date().setDate(new Date().getDate() - 1));
is.yesterday(yesterday);
=> true
is.not.yesterday(today);
=> true
is.all.yesterday(yesterday, today);
=> false
is.any.yesterday(today, yesterday);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.yesterday([today, yesterday]);
=> false
is.tomorrow(value:object)
interfaces: not, all, anyChecks if the given date object indicate tomorrow.
var today = new Date();
is.tomorrow(today);
=> false
var tomorrow = new Date(new Date().setDate(new Date().getDate() + 1));
is.tomorrow(tomorrow);
=> true
is.not.tomorrow(today);
=> true
is.all.tomorrow(tomorrow, today);
=> false
is.any.tomorrow(today, tomorrow);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.tomorrow([today, tomorrow]);
=> false
is.past(value:object)
interfaces: not, all, anyChecks if the given date object indicate past.
var yesterday = new Date(new Date().setDate(new Date().getDate() - 1));
var tomorrow = new Date(new Date().setDate(new Date().getDate() + 1));
is.past(yesterday);
=> true
is.past(tomorrow);
=> false
is.not.past(tomorrow);
=> true
is.all.past(tomorrow, yesterday);
=> false
is.any.past(yesterday, tomorrow);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.past([yesterday, tomorrow]);
=> false
is.future(value:object)
interfaces: not, all, anyChecks if the given date object indicate future.
var yesterday = new Date(new Date().setDate(new Date().getDate() - 1));
var tomorrow = new Date(new Date().setDate(new Date().getDate() + 1));
is.future(yesterday);
=> false
is.future(tomorrow);
=> true
is.not.future(yesterday);
=> true
is.all.future(tomorrow, yesterday);
=> false
is.any.future(yesterday, tomorrow);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.future([yesterday, tomorrow]);
=> false
is.day(value:object, dayString)
interface: notChecks if the given date objects' day equal given dayString parameter.
var mondayObj = new Date('01/26/2015');
var tuesdayObj = new Date('01/27/2015');
is.day(mondayObj, 'monday');
=> true
is.day(mondayObj, 'tuesday');
=> false
is.not.day(mondayObj, 'tuesday');
=> true
is.month(value:object, monthString)
interface: notChecks if the given date objects' month equal given monthString parameter.
var januaryObj = new Date('01/26/2015');
var februaryObj = new Date('02/26/2015');
is.month(januaryObj, 'january');
=> true
is.month(februaryObj, 'january');
=> false
is.not.month(februaryObj, 'january');
=> true
is.year(value:object, yearNumber)
interface: notChecks if the given date objects' year equal given yearNumber parameter.
var year2015 = new Date('01/26/2015');
var year2016 = new Date('01/26/2016');
is.year(year2015, 2015);
=> true
is.year(year2016, 2015);
=> false
is.not.year(year2016, 2015);
=> true
is.leapYear(value:number)
interfaces: not, all, anyChecks if the given year number is leapYear.
is.leapYear(2016);
=> true
is.leapYear(2015);
=> false
is.not.leapYear(2015);
=> true
is.all.leapYear(2015, 2016);
=> false
is.any.leapYear(2015, 2016);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.leapYear([2016, 2080]);
=> true
is.weekend(value:object)
interfaces: not, all, anyChecks if the given date objects' day is weekend.
var monday = new Date('01/26/2015');
var sunday = new Date('01/25/2015');
var saturday = new Date('01/24/2015');
is.weekend(sunday);
=> true
is.weekend(monday);
=> false
is.not.weekend(monday);
=> true
is.all.weekend(sunday, saturday);
=> true
is.any.weekend(sunday, saturday, monday);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.weekend([sunday, saturday, monday]);
=> false
is.weekday(value:object)
interfaces: not, all, anyChecks if the given date objects' day is weekday.
var monday = new Date('01/26/2015');
var sunday = new Date('01/25/2015');
var saturday = new Date('01/24/2015');
is.weekday(monday);
=> true
is.weekday(sunday);
=> false
is.not.weekday(sunday);
=> true
is.all.weekday(monday, saturday);
=> false
is.any.weekday(sunday, saturday, monday);
=> true
// 'all' and 'any' interfaces can also take array parameter
is.all.weekday([sunday, saturday, monday]);
=> false
is.inDateRange(value:object, startObject, endObject)
interface: notChecks if the date is within given range.
var saturday = new Date('01/24/2015');
var sunday = new Date('01/25/2015');
var monday = new Date('01/26/2015');
is.inDateRange(sunday, saturday, monday);
=> true
is.inDateRange(saturday, sunday, monday);
=> false
is.not.inDateRange(saturday, sunday, monday);
=> true
is.inLastWeek(value:object)
interface: notChecks if the given date is between now and 7 days ago.
var twoDaysAgo = new Date(new Date().setDate(new Date().getDate() - 2));
var nineDaysAgo = new Date(new Date().setDate(new Date().getDate() - 9));
is.inLastWeek(twoDaysAgo);
=> true
is.inLastWeek(nineDaysAgo);
=> false
is.not.inLastWeek(nineDaysAgo);
=> true
is.inLastMonth(value:object)
interface: notChecks if the given date is between now and a month ago.
var tenDaysAgo = new Date(new Date().setDate(new Date().getDate() - 10));
var fortyDaysAgo = new Date(new Date().setDate(new Date().getDate() - 40));
is.inLastMonth(tenDaysAgo);
=> true
is.inLastMonth(fortyDaysAgo);
=> false
is.not.inLastMonth(fortyDaysAgo);
=> true
is.inLastYear(value:object)
interface: notChecks if the given date is between now and a year ago.
var twoMonthsAgo = new Date(new Date().setMonth(new Date().getMonth() - 2));
var thirteenMonthsAgo = new Date(new Date().setMonth(new Date().getMonth() - 13));
is.inLastYear(twoMonthsAgo);
=> true
is.inLastYear(thirteenMonthsAgo);
=> false
is.not.inLastYear(thirteenMonthsAgo);
=> true
is.inNextWeek(value:object)
interface: notChecks if the given date is between now and 7 days later.
var twoDaysLater = new Date(new Date().setDate(new Date().getDate() + 2));
var nineDaysLater = new Date(new Date().setDate(new Date().getDate() + 9));
is.inNextWeek(twoDaysLater);
=> true
is.inNextWeek(nineDaysLater);
=> false
is.not.inNextWeek(nineDaysLater);
=> true
is.inNextMonth(value:object)
interface: notChecks if the given date is between now and a month later.
var tenDaysLater = new Date(new Date().setDate(new Date().getDate() + 10));
var fortyDaysLater = new Date(new Date().setDate(new Date().getDate() + 40));
is.inNextMonth(tenDaysLater);
=> true
is.inNextMonth(fortyDaysLater);
=> false
is.not.inNextMonth(fortyDaysLater);
=> true
is.inNextYear(value:object)
interface: notChecks if the given date is between now and a year later.
var twoMonthsLater = new Date(new Date().setMonth(new Date().getMonth() + 2));
var thirteenMonthsLater = new Date(new Date().setMonth(new Date().getMonth() + 13));
is.inNextYear(twoMonthsLater);
=> true
is.inNextYear(thirteenMonthsLater);
=> false
is.not.inNextYear(thirteenMonthsLater);
=> true
is.quarterOfYear(value:object, quarterNumber)
interface: notChecks if the given date is in the parameter quarter.
var firstQuarter = new Date('01/26/2015');
var secondQuarter = new Date('05/26/2015');
is.quarterOfYear(firstQuarter, 1);
=> true
is.quarterOfYear(secondQuarter, 1);
=> false
is.not.quarterOfYear(secondQuarter, 1);
=> true
is.dayLightSavingTime(value:object, quarterNumber)
interface: notChecks if the given date is in daylight saving time.
// For Turkey Time Zone
var january1 = new Date('01/01/2015');
var june1 = new Date('06/01/2015');
is.dayLightSavingTime(june1);
=> true
is.dayLightSavingTime(january1);
=> false
is.not.dayLightSavingTime(january1);
=> true
configuration methods
is.setRegexp(value:RegExp, regexpString)
Override RegExps if you think they suck.
is.url('https://www.duckduckgo.com');
=> true
is.setRegexp(/quack/, 'url');
is.url('quack');
=> true
is.setNamespace()
Change namespace of library to prevent name collisions.
var customName = is.setNamespace();
customName.odd(3);
// => true
is.socialSecurityNumber(value:any)
interfaces: not, all, anyChecks if the given value matches social security number regexp.